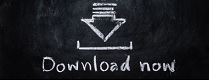
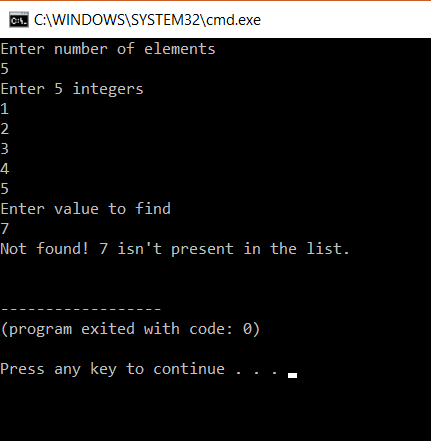
We first search for the element and if it is not found at the required place (where it should be) then we just insert a new node at that position. Inserting a new node is similar to searching for an element in a tree. Root->left_child = insert(root->left_child,x) Root->right_child = insert(root->right_child, x) Struct node* insert(struct node *root, int x) If(root=NULL || root->data=x) → If the null value is reached then the element is not in the tree and if the data at the root is equal to x then the element is found.Įlse if(x>root->data) → The element is greater than the data at the root, so we will search in the right subtree – search(root->right_child, x). Otherwise, on the left subtree – search(root->left_child,x). We repeat this process until the element is found or a null value is reached (the element is not in the tree). To search an element we first visit the root and if the element is not found there, then we compare the element with the data of the root and if the element is greater, then it must lie on the right subtree (property of a BST – All elements greater than the data at the node are on the right subtree), otherwise on the left subtree. Search is a function to find any element in the tree. Struct node* search(struct node *root, int x) Also, the concepts behind a binary search tree are explained in the post Binary Search Tree. Here, we will focus on the parts related to the binary search tree like inserting a node, deleting a node, searching, etc. The making of a node and traversals are explained in the post Binary Trees in C: Linked Representation & Traversals. This allows the application to easily search for database rows by specifying a key, for example, to find a user record using the email primary key.#include #include struct node Explanation
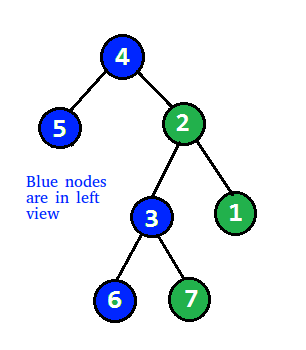
There are many applications of binary search trees in real life, and one of the most common use cases is storing indexes and keys in a database.įor example, when you create a primary key column in MySQL or PostgresQL, you create a binary tree where the keys are the values of the column and the nodes point to database rows. For example, getting a user record by the email primary key.
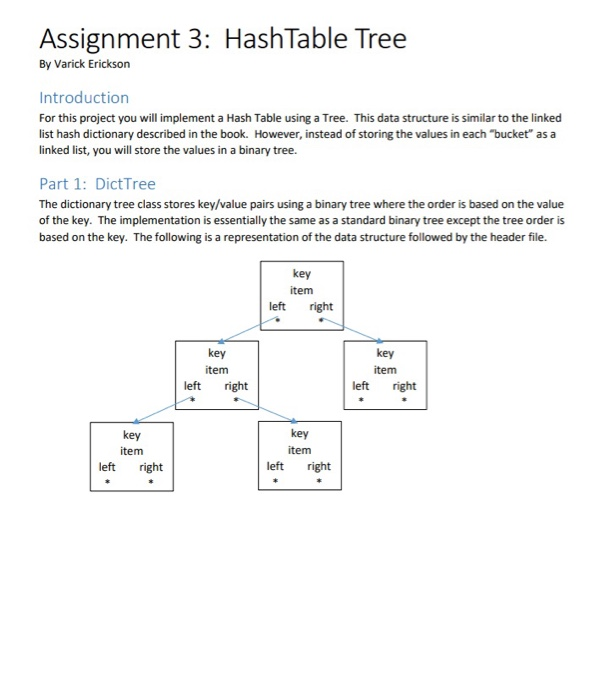
This lets the application easily search database rows by providing a key. There are many applications of binary search trees in real life, and one of the most common use-cases is in storing indexes and keys in a database.įor example, in MySQL or PostgresQL when you create a primary key column, what you’re really doing is creating a binary tree where the keys are the values of the column, and those nodes point to database rows. Where would you use a binary search tree in real life? The exists function is another simple recursive function that returns True or False depending on whether a given value already exists in the tree. This allows a parent whose child has been deleted to properly set it’s left or right data member to None. It is a recursive function as well, but it also returns the new state of the given node after performing the delete operation. The delete operation is one of the more complex ones. Since pointers to whole objects are typically involved, a BST can require quite a bit more memory than an array, although this depends on the implementation.When the tree becomes unbalanced, all fast O(log(n)) operations quickly degrade to O(n).If you need to iterate over each node, you might have more success with an array. An ordinary BST, unlike a balanced tree like a red-black tree, requires very little code to get running. Binary search trees are pretty simple.When balanced, a BST provides lightning-fast O(log(n)) insertions, deletions, and lookups.Many popular production databases such as PostgreSQL and MySQL use binary trees under the hood to speed up CRUD operations. Log(n) is much faster than the linear O(n) time required to find elements in an unsorted array. To be precise, binary search trees provide an average Big-O complexity of O(log(n)) for search, insert, update, and delete operations. This arrangement of nodes lets each comparison skip about half of the rest of the tree, so the entire search is lightning fast. Writing a Binary Search Tree in Python with ExamplesĪ binary search tree, or BST for short, is a tree whose nodes store a key that is greater than all of their left child nodes and less than all of their right child nodes.īinary trees are useful for storing data in an organized manner so that it can be quickly retrieved, inserted, updated, and deleted.
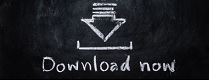